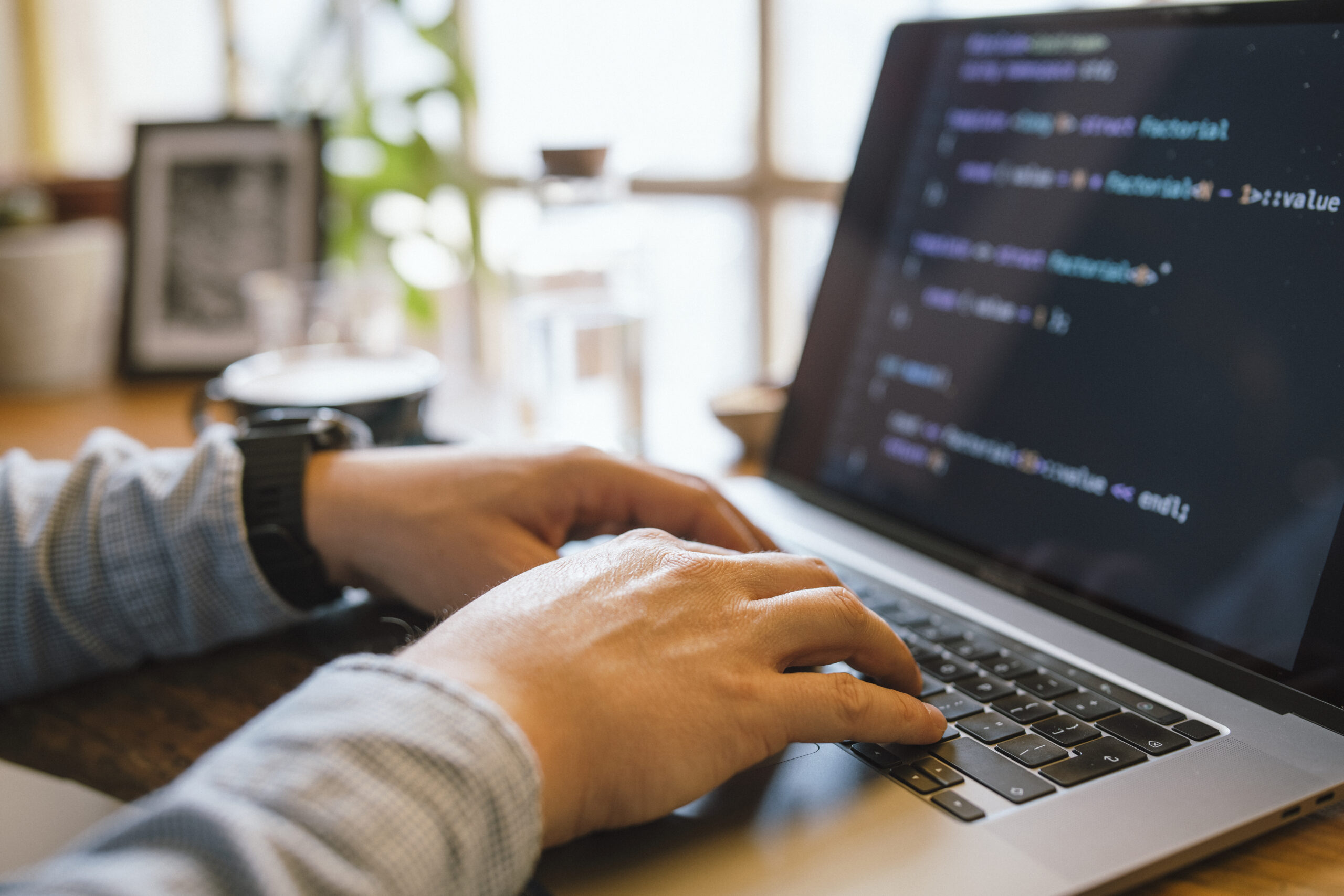
Debugging is one of the most vital — nonetheless frequently disregarded — capabilities in a very developer’s toolkit. It's not just about fixing broken code; it’s about comprehension how and why points go Completely wrong, and learning to Believe methodically to solve issues effectively. Regardless of whether you're a novice or even a seasoned developer, sharpening your debugging capabilities can preserve hrs of disappointment and substantially increase your productiveness. Allow me to share many approaches to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Applications
On the list of fastest approaches developers can elevate their debugging abilities is by mastering the tools they use every day. Though producing code is one particular Portion of improvement, recognizing ways to communicate with it successfully for the duration of execution is equally important. Fashionable progress environments arrive equipped with highly effective debugging abilities — but a lot of developers only scratch the area of what these instruments can perform.
Get, as an example, an Built-in Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools assist you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code about the fly. When used effectively, they let you notice exactly how your code behaves through execution, that is a must have for tracking down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for entrance-conclude builders. They let you inspect the DOM, monitor community requests, see authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can convert aggravating UI difficulties into manageable duties.
For backend or process-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Management around operating procedures and memory administration. Learning these resources could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, come to be comfortable with Edition Management devices like Git to know code background, uncover the precise minute bugs were being released, and isolate problematic modifications.
In the end, mastering your equipment signifies likely further than default configurations and shortcuts — it’s about developing an intimate knowledge of your advancement setting making sure that when challenges crop up, you’re not lost at midnight. The better you realize your resources, the more time you'll be able to devote fixing the actual difficulty as opposed to fumbling by way of the method.
Reproduce the challenge
The most essential — and sometimes disregarded — measures in successful debugging is reproducing the trouble. Just before jumping into the code or earning guesses, builders need to have to create a consistent environment or state of affairs where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a activity of possibility, usually leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as possible. Talk to inquiries like: What actions resulted in the issue? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it gets to be to isolate the precise circumstances less than which the bug happens.
As you’ve gathered enough facts, attempt to recreate the condition in your local ecosystem. This could necessarily mean inputting the identical data, simulating related user interactions, or mimicking technique states. If the issue appears intermittently, take into consideration composing automatic tests that replicate the sting conditions or state transitions included. These tests not merely assistance expose the trouble and also prevent regressions Later on.
In some cases, the issue could possibly be ecosystem-particular — it would materialize only on particular working units, browsers, or below distinct configurations. Working with tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is usually instrumental in replicating such bugs.
Reproducing the challenge isn’t simply a step — it’s a state of mind. It calls for tolerance, observation, in addition to a methodical solution. But when you can persistently recreate the bug, you happen to be now midway to correcting it. With a reproducible scenario, You can utilize your debugging equipment much more successfully, check likely fixes safely and securely, and talk far more Plainly with the staff or people. It turns an summary grievance into a concrete challenge — and that’s exactly where developers prosper.
Browse and Understand the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when anything goes Mistaken. As opposed to seeing them as frustrating interruptions, builders really should understand to deal with error messages as immediate communications with the system. They often show you what precisely took place, in which it occurred, and occasionally even why it happened — if you know the way to interpret them.
Start off by reading through the message carefully As well as in entire. Several developers, specially when beneath time pressure, look at the initial line and immediately get started generating assumptions. But deeper from the error stack or logs may possibly lie the accurate root induce. Don’t just copy and paste mistake messages into serps — go through and understand them 1st.
Break the mistake down into parts. Could it be a syntax error, a runtime exception, or possibly a logic error? Does it point to a certain file and line quantity? What module or purpose triggered it? These issues can tutorial your investigation and stage you towards the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Understanding to acknowledge these can significantly hasten your debugging process.
Some problems are imprecise or generic, As well as in Individuals scenarios, it’s essential to examine the context in which the error transpired. Look at associated log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede more substantial challenges and provide hints about prospective bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, serving to you pinpoint issues quicker, lessen debugging time, and turn into a additional economical and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When applied proficiently, it offers authentic-time insights into how an software behaves, encouraging you understand what’s going on underneath the hood without having to pause execution or move in the code line by line.
A good logging strategy begins with being aware of what to log and at what amount. Frequent logging stages involve DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for thorough diagnostic facts through progress, Details for common functions (like prosperous get started-ups), Alert for likely concerns that don’t break the appliance, ERROR for precise challenges, and Deadly once the method can’t continue.
Avoid flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure important messages and decelerate your program. Concentrate on vital gatherings, state changes, enter/output values, and critical conclusion factors inside your code.
Structure your log messages Plainly and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to track how variables evolve, what ailments are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re especially worthwhile in production environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with monitoring dashboards.
Finally, smart logging is about equilibrium and clarity. Having a very well-thought-out logging strategy, you may reduce the time it requires to identify issues, achieve further visibility into your applications, and improve the Over-all maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not just a technical process—it is a form of investigation. To efficiently discover and take care of bugs, developers should technique the procedure like a detective solving a thriller. This mentality helps break down sophisticated difficulties into workable pieces and adhere to clues logically to uncover the root result in.
Start off by accumulating proof. Think about the signs and symptoms of the challenge: mistake messages, incorrect output, or effectiveness challenges. Identical to a detective surveys against the law scene, collect just as much applicable information as you can with out jumping to conclusions. Use logs, test cases, and user reports to piece together a transparent photograph of what’s occurring.
Following, kind hypotheses. Question by yourself: What could possibly be leading to this conduct? Have any improvements not long ago been made for the codebase? Has this situation transpired just before beneath equivalent situations? The objective is to narrow down choices and detect opportunity culprits.
Then, exam your theories systematically. Endeavor to recreate the situation in a very managed setting. Should you suspect a particular perform or ingredient, isolate it and verify if the issue persists. Similar to a detective conducting interviews, request your code concerns and Enable the outcome lead you nearer to the truth.
Spend close awareness to smaller information. Bugs usually disguise while in the least envisioned areas—like a lacking semicolon, an off-by-1 mistake, or even a race condition. Be extensive and affected person, resisting the urge to patch The difficulty without having totally knowledge it. Short-term fixes may perhaps conceal the actual difficulty, just for it to resurface later.
And finally, keep notes on Whatever you tried and realized. Equally as detectives log their investigations, documenting your debugging method can help save time for long term difficulties and help Other folks understand your reasoning.
By pondering just like a detective, builders can sharpen their analytical abilities, technique complications methodically, and become more effective at uncovering hidden difficulties in complex techniques.
Publish Checks
Writing tests is one of the best solutions to improve your debugging abilities and All round progress performance. Checks not only assist catch bugs early but in addition function a security Internet that offers you assurance when earning changes for your codebase. A effectively-examined application is easier to debug since it permits you to pinpoint just the place and when a challenge happens.
Begin with unit exams, which give attention to personal functions or modules. These little, isolated tests can rapidly reveal whether or not a specific piece of logic is working as envisioned. Every time a take a look at fails, you promptly know wherever to glance, appreciably cutting down enough time put in debugging. Unit tests are Primarily practical for catching regression bugs—challenges that reappear immediately after Earlier currently being set.
Next, combine integration assessments and stop-to-finish tests into your workflow. These enable be certain that different parts of your software get the job done collectively smoothly. They’re significantly valuable for catching bugs that take place in complex units with a number of elements or products and services interacting. If a thing breaks, your exams can show you which Section of the pipeline failed and less than what situations.
Writing assessments also forces you to Assume critically about your code. To check a characteristic correctly, you would like to grasp its inputs, expected outputs, and edge situations. This level of comprehension Normally potential customers to better code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the check fails continually, you'll be able to deal with repairing the bug and watch your examination go when the issue is settled. This tactic ensures that the identical bug doesn’t return Down the road.
In short, creating checks turns debugging from a annoying guessing activity into a structured and predictable procedure—supporting you capture extra bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s straightforward to become immersed in the situation—gazing your screen for hours, attempting Remedy immediately after Alternative. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and often see the issue from the new standpoint.
If you're much too near the code for much too long, cognitive exhaustion sets in. You might start overlooking noticeable faults or misreading code that you choose to wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee break, or perhaps switching to a different endeavor for ten–quarter-hour can refresh your concentration. A lot of developers report discovering the foundation of a challenge once they've taken time for you to disconnect, permitting their subconscious do the job from the track record.
Breaks also assist prevent burnout, Primarily through for a longer time debugging sessions. Sitting down in front of a screen, mentally trapped, is not just unproductive but also draining. Stepping absent permits you to return with renewed energy in addition to a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, an excellent rule of thumb is usually to set a timer—debug actively for forty five–sixty minutes, then take a five–10 moment break. Use that point to maneuver all around, stretch, or do anything unrelated to code. It may experience counterintuitive, Specially under restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, using breaks will not be a sign of weak point—it’s a sensible technique. It offers your Mind Area to breathe, enhances your standpoint, and aids you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of fixing it.
Learn From Each and every Bug
Each individual bug you experience is much more than simply A short lived setback—It is a chance to improve as a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can more info instruct you something beneficial in case you go to the trouble to reflect and evaluate what went Mistaken.
Get started by inquiring your self a number of critical thoughts once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with greater techniques like device tests, code opinions, or logging? The responses normally expose blind places in the workflow or understanding and assist you to Develop stronger coding routines shifting forward.
Documenting bugs can also be an excellent habit. Keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you uncovered. With time, you’ll start to see patterns—recurring issues or popular issues—you could proactively prevent.
In staff environments, sharing Whatever you've realized from a bug with all your friends might be Specifically potent. Whether it’s via a Slack concept, a brief produce-up, or a quick understanding-sharing session, assisting others steer clear of the very same problem boosts workforce performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your way of thinking from stress to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. In spite of everything, a number of the best builders aren't those who create fantastic code, but individuals who continuously understand from their errors.
In the long run, Every bug you deal with adds a whole new layer to your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear away a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills normally takes time, observe, and patience — even so the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.